Functions Beacon
The Functions Beacon is a private, permissionless smart contract built on top of Chainlink Functions that makes interacting with the Functions decentralized oracle network easy, repeatable, and more cost effective.
Primary vs. Secondary Functions Consumer
Functions Consumers are smart contracts that are enabled to request computation from the decentralized oracle network.
Primary Functions Consumer:
- Directly interacts with Chainlink's decentralized oracle network (DON)
- Must manage its own Functions subscription
- Handle callbacks from oracles themselves
- Consumer pays for compute from subscription-level LINK balance
Secondary Functions Consumer:
- Interact through an intermediary contract (the Chainlink Functions Beacon)
- Pay on a per-transaction basis using native token not LINK subscription
- Receives callbacks through the intermediary client (the primary consumer)
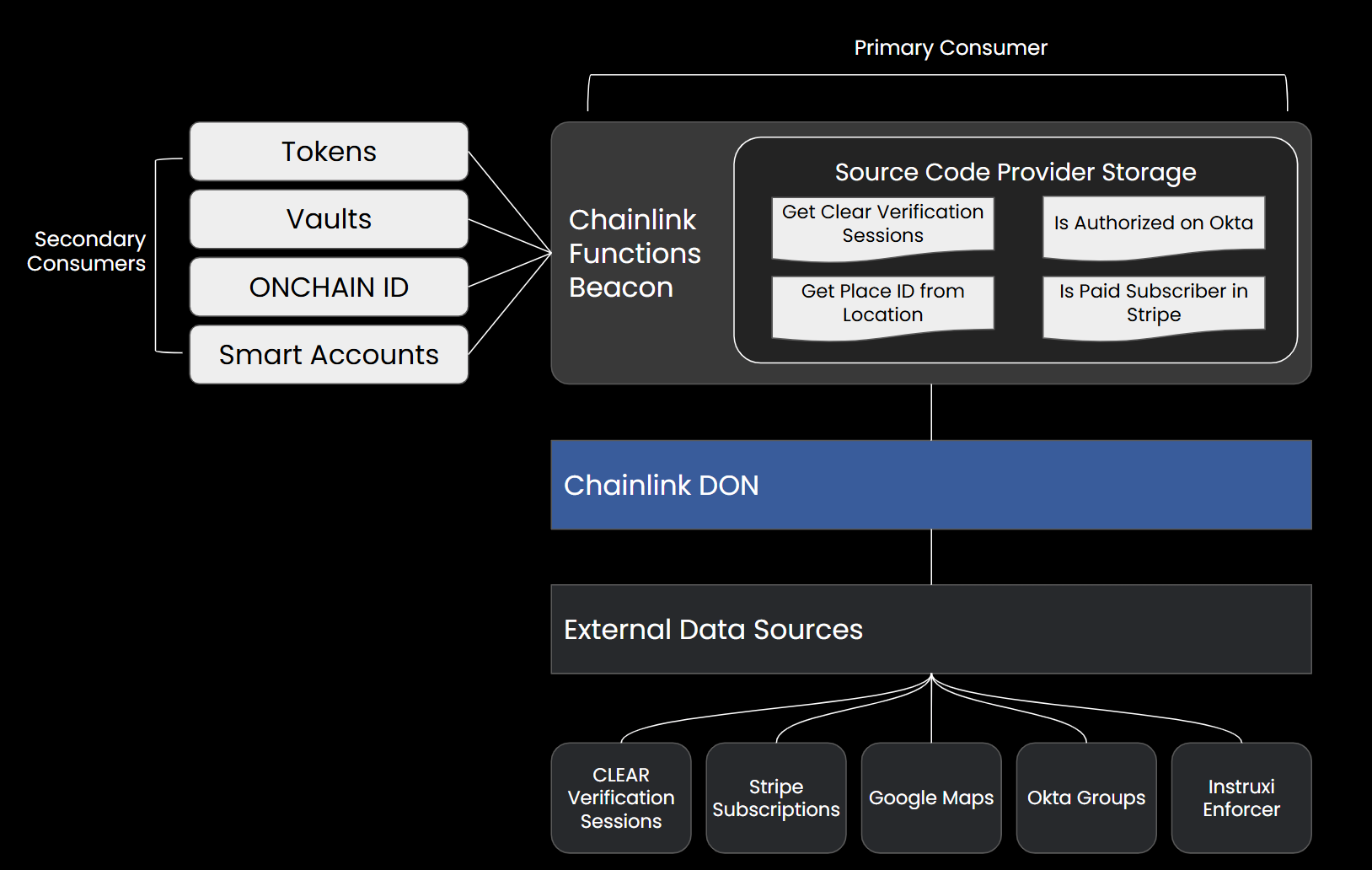
In the scenario shown above, the Chainlink Functions Beacon contract, operated by Instruxi, is acting as the Primary Consumer. The arbitrary contracts shown on the far left are authorized on the Beacon and act as Secondary Consumers to the oracle network
Name | Description | Type |
---|---|---|
Functions Beacon | Acts as a Primary Consumer smart contract for Chainlink Functions and is the entry point to the Source Code library. Secondary Consumers may be added to this contracts auth list | Primary Consumer |
Mesh ID | Soulbound ERC1155 smart contract that represents your user account's latest verification session as a token with configurable thresholds | Secondary Consumer |
Location Indexer | ERC721 smart contract that represents global location as a token given a latitude and longitude | Secondary Consumer |
Getting Started
This tutorial walks through the example usage Hardhat project here. The purpose of the repo is to demonstrate developers can contribute Functions in the form of source code on the Beacon smart contract as well as show users how to build smart contracts that act as secondary consumers to the DON network.
Prerequisites
- Ethereum development environment (Hardhat)
- Web3 wallet with test net native token to pay for gas
- Basic Solidity knowledge
Integration Steps (Hardhat)
- Clone Starter Project
git clone
- Install Dependencies
npm install
- Build and Deploy a Beacon Client contract
Your contract must implement the callback function and must be authorized on the Beacon contract by an Admin:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
/**
* @title SimpleBeaconClient
* @dev A client contract that interacts with a beacon oracle
*/
contract MinimalBeaconClient {
/**
* @notice Initiates a computation request to the beacon oracle
* @dev Sends encoded data to the beacon for processing
*/
function makeRequest() external {
bytes32 requestId = beacon.requestComputation(
data, // Your encoded request data
source // Source code provider hash
);
}
/**
* @notice Callback function invoked by the beacon oracle with computation results
* @param requestId Unique identifier for the request
* @param response Response data from the computation
* @param err Error message if the computation failed
*/
function oracleCallback(
bytes32 requestId,
bytes memory response,
bytes memory err
) external onlyBeacon {
// Callback implementation
}
}
Updated about 13 hours ago